52. Search a 2D Matrix
Topic :
binary search
Difficulty :
medium
Problem Link :
problem statement
You are given an m x n
integer matrix matrix
with the following two properties:
- Each row is sorted in non-decreasing order.
- The first integer of each row is greater than the last integer of the previous row.
Given an integer target
, return true
if target
is in matrix
or false
otherwise.
You must write a solution in O(log(m * n))
time complexity.
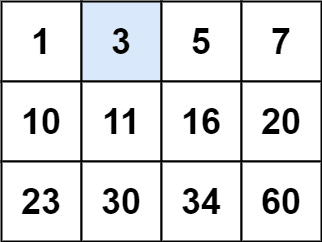
Example 1:
Input: matrix = [[1,3,5,7],[10,11,16,20],[23,30,34,60]],
target = 3
Output: true
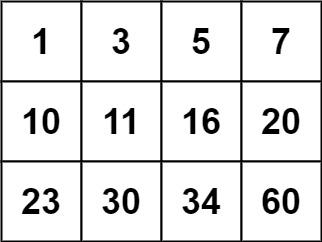
Example 2:
Input: matrix = [[1,3,5,7],[10,11,16,20],[23,30,34,60]],
target = 13
Output: false
Constraints:
m == matrix.length
n == matrix[i].length
1 <= m, n <= 100
-104 <= matrix[i][j], target <= 104
solution
import java.io.*;
import java.util.*;
class Search_in_a_2D_Matrix
{
public static void main (String args[]){
int [][] matrix = {{1,3,5,7},{10,11,16,20},{23,30,34,60}};
int target = 3;
System.out.println(searchMatrix(matrix,target));
}
static boolean searchMatrix(int[][] matrix, int target) {
if(matrix.length==0||matrix==null)
return false;
int n=matrix.length;
int m=matrix[0].length;
int low=0;
int high=(n*m)-1;
while (low<=high)
{
int mid=(low+high)/2;
if(matrix[mid/m][mid%m]==target)
return true;
if(matrix[mid/m][mid%m]<target)
low=mid+1;
else
high=mid-1;
}
return false;
}
}